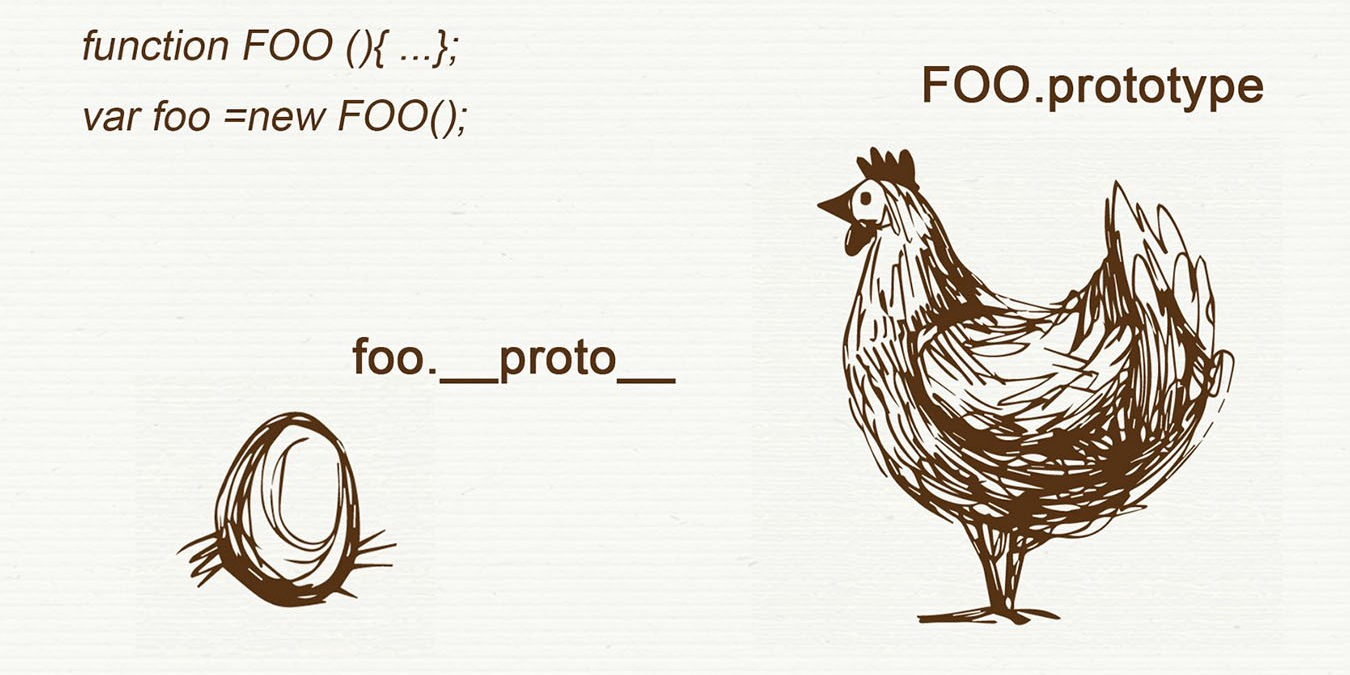
What is a prototype?
Prototype is the property on a function that is set as property if you are using the new
keyword.
Definition of __proto__
__proto__
is the property on an object that points out the prototype that has been set out for that object
Let’s have a look at an example to get some clarity:
Example 1:
Lets say you had to type in the following in your browser console.
function Dog() {};
//Then type
Dog.prototype;
There will automatically be an object created for that function.

However, if you had to create a basic object literal
like giraffe
let Giraffe = {};
//Then type
Giraffe.prototype;
Giraffe.prototype would be undefined. Hence going back to the definition of prototype. “A property on a function
…”
__proto__
is added to constructor when the keyword new
is used. It essentially acts as a reference point so that the constructor can know which prototype to reference.
if we create a dog constructor
function Dog() {};
Dog.proto.breed = "Bulldog";
Then create a myDoggie constructor
let myDoggie = new Dog();
My myDoggie will have a __proto__
that
references the prototype of Dog.prototype

If we can a new attribute to the prototype like color
, __proto__
will reference the updated prototype
Dog.prototype.color = "maroon";
myDoggie
's __proto__
will equal Dog.prototype
Anyways, that's all for today. Happy coding!