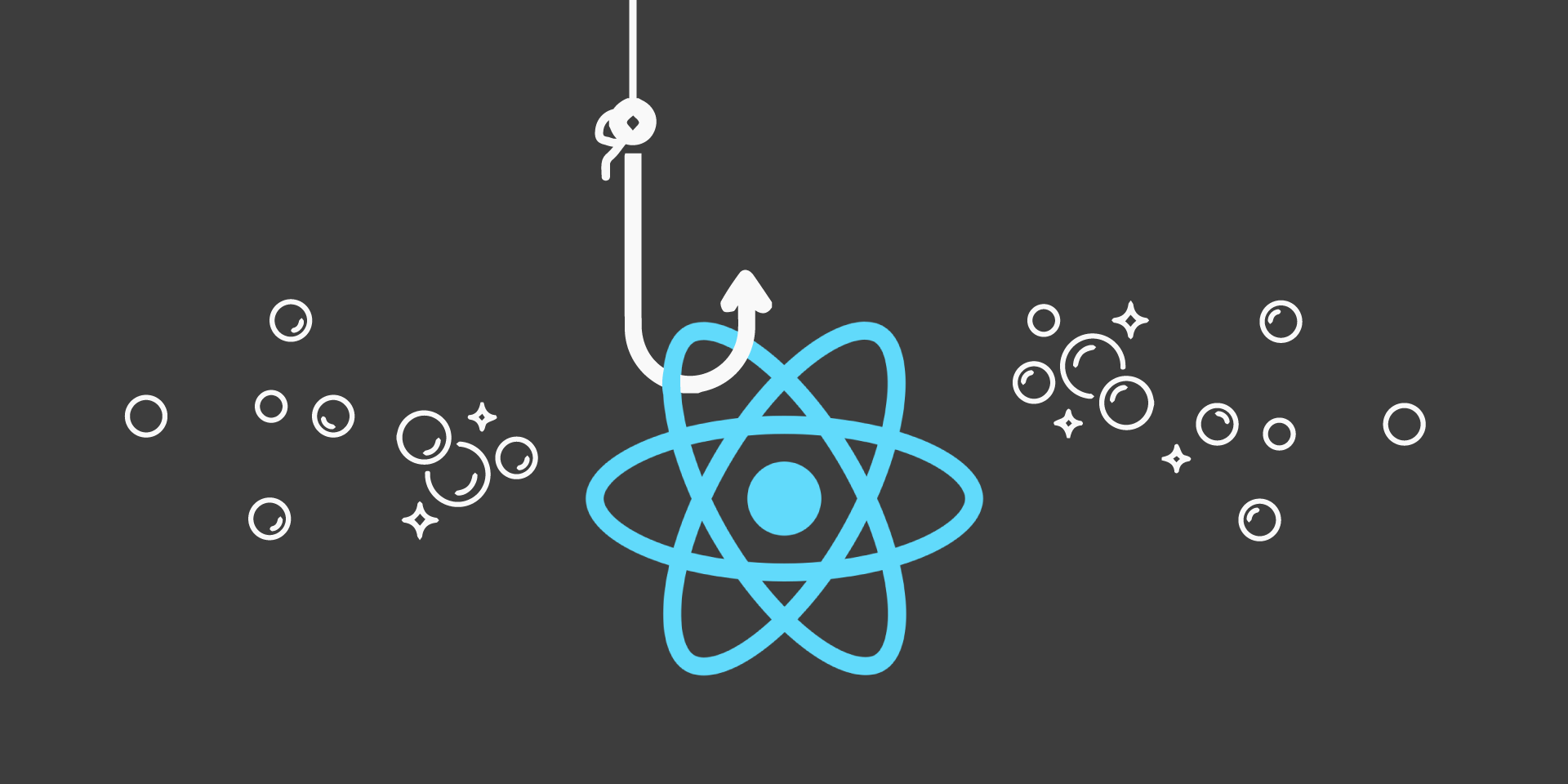
Get Hooked
The purpose of this article is give an introduction into the wonderful world of hooks.
Article Outline
- What are react hooks?
- Why you should consider using them for future development?
- Giving an explanation on the two most common hooks used: useState and useEffect
- Briefly mention other hooks that exist
- Github repo with basic examples
What is a react hook?
Hooks are functions that let you “hook into” React state and lifecycle features from function components.
Why should we use react hooks?
1) Class based components can become very complicated very quickly
What starts out as a very simple component can become a very messy component due to stateful logic and side effectsWhat generally happens is that each life cycle contains unrelated logic
Example: Class Based Component
In a class component, it could have ComponentDidMount which sets up an event listener and code which sets the DOM like document.title according to the current state.Then have componentDidUpdate which updates document.title every the state updatesThen have clean up code for the event listener in componentWillUnmount.
The problem with this
- Mutually related code that changes together gets split apart
- Completely unrelated code ends up combined in a single method could lead to many inconsistencies and bugs in the component
- It becomes hard to test because stateful logic is over the place
- How hooks can solve this problem
- Hooks allow you to separate a component into functions based on what pieces are related
2) Classes cause confusion for people and machines
Lets start with humans:he learning curve can be quite steep due to the following concepts:
- Javascript's this
- The usage of binding event handler and knowing when to do so
- When to use class components and when to use function components. (This can cause debates among experienced devs)
Component Folding:
- Class components dont minify very well and they cause hotloading to be unreliable
- Functional components have to seen to bundle very well with Prepack.
- Hooks solve this problem by allowing you to use React's features in functional component
Types React Hooks
useState:
- It is a hook that can be called inside a function component to add some local state to it
- it preserves state between renders
- returns a tuple: the current state value and a function that lets you update the state (somewhat similar to this.setState)
- useState has one argument which is initial state which can also accept a function
import React, { useState } from "react";
function Hipsters() {
// Declare a new state variable, which we'll call "count"
const [hipsterCount, addAHipster] = useState(0);
return (
<div>
<p>You clicked {hipsterCount} times</p>
<button onClick={() => addAHipster(hipsterCount + 1)}>Click me</button>
</div>
);
}
useEffect:
Before getting into useEffect we need to understand what side effects are:
Explanation on Side Effects:
- Data fetching, configuring a subscription and or manually changing a DOM in React components are all examples of side effects.
- There are two kinds of sideEffects: those that do not require cleanup and those that do
Explanation on useEffect:
- Simply put, the useEffect hook tells react to do something after your component renders
- useEffect adds the ability to perform side effects from a functional component. serving a purpose of componentDidMount, componentDidUpdate, componentWillUnmount in one single api
The cleanup part of useEffect:
- So as we know the purpose of a clean up is to undo effect for cases like an event listener
- React only runs effects after letting the browser paint
- Most of the effects will not need block screen updates resulting in faster apps
- The previous effect is cleaned up after the re-render with new props
Other Hooks to be aware of
useRef:
It is a pseudo equivalent to createRef which exist in class based components. The useRef Hook is a function that returns a mutable ref object whose .current property is initialized to the passed argument (initialValue). The returned object will persist for the full lifetime of the component.
i.e
function TextInputWithFocusButton() {
const inputEl = useRef(null);
const onButtonClick = () => {
// `current` points to the mounted text input element
inputEl.current.focus();
};
return (
<>
<input ref={inputEl} type="text" />
<button onClick={onButtonClick}>Focus the input</button>
</>
);
}
useReducer:
Accepts a reducer of type (state, action) => newState, and returns the current state tuple with a dispatch method.
i.e
const [state, dispatch] = useReducer(reducer, initialState);
useContext:
Its a hook that allows you to access the state from the context instead of using Context.Consumer as a wrapper component.
I made a repo fulled with basic examples to reference
Happy coding!